Uberzoik
| |
Note: what appears here is what I prepared as a project for
the "Computer Graphics I" course, which I attended at
the University of Illinois at Chicago (course number EECS488) with
Andrew Johnson as instructor.
|
Overview
|
Uberzoik is an improvement over Berzoik,
being a walkable three-dimensional maze with the addition of
texture mapping, lighting, fog and other features.
Sorry, there is still nothing ready to download.
|
Screen shots
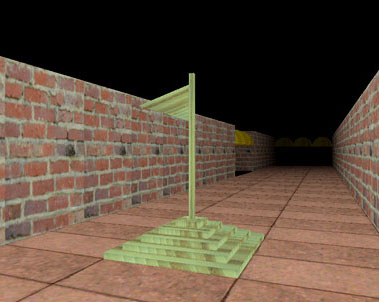
Specifications
|
The official specifications of the assignment, as taken from the professor's website on October 2000.
It should explain pretty well what the program does.
EECS 488: Assignment 4
'Uberzoik'
Out: 11/7/00
Due: 11/28/00 at 3:00pm
In this fourth assignment you will enhance the program you wrote in assignment 3 and experiment with some of the more advanced features of OpenGL.
This assignment will be a little different in that I'm hoping its closer to the 'real world.' I'm going to tell you what features the application should have, and some of the commands you will need, but for several of the features you will need to dig into the red book and learn by doing.
Your program will read in a datafile called maze.txt which has _almost_ the same format as the one from the previous assignment. The maze will contain the same objects but this time you will create two new interesting objects called A and B which should be of your own design and purpose.
This enhanced version adds the following to the requirements given in assignment 3:
- You will draw everything with filled polygons rather than just lines. Instead of using with glBegin(GL_LINE_LOOP); you should look at GL_POLYGON.
- You will use smooth shading (rather than flat shading) for all of the polygons with
glShadeModel(GL_SMOOTH);
- You will set up window to have a depth buffer using
glutInitDisplayMode(GLUT_RGBA | GLUT_DOUBLE | GLUT_DEPTH);
and enable the Z-buffer with
glEnable(GL_DEPTH_TEST);
and at the beginning of each draw cycle you will need to clear the depth buffer as well as the colour buffer with
glClear(GL_DEPTH_BUFFER_BIT | GL_COLOR_BUFFER_BIT);
so that occluded polygons are not drawn
- You will turn on back-face culling with
glCullFace(GL_BACK);
glEnable(GL_CULL_FACE);
so only the appropriate sides of the polygons show up. Note that when drawing with lines that the order the vertices were specified in did not matter, but here it will matter - with back-face culling turned on the polygons must be drawn in counterclockwise order when seen from their visible side or they won't show up.
- Instead of defining the colour of the lines as in assignment 3, here you will define the material properties of the polygons. This gives you much more control over how objects appear but for this assignment we will only deal with the diffuse colour of each polygon. You will be using glMaterialfv(GL_FRONT, GL_DIFFUSE, <your properties vector>);
- The user will carry a white candle (point light) to illuminate the objects in the maze. That is you will need to define an appropriate white point light source that moves with the player through the scene.
glEnable(GL_LIGHTING);
glEnable(GL_LIGHT0);
and you will need to define some parameters for the light source itself e.g. its position. The light's position will have a w value of 1
- Since we are using lighting we should also define the normals for each vertex as we define the object using glNormal3f(nx,ny,nz); This means we need some way to define what the normals are - so we are going to modify the data files for the program slightly. In between the number of vertices and the first vertex definition we are going to insert a line giving the vertex for the normal e.g.
4
0 1 0 <--- new line giving the normal for all of the vertices in this polygon
-2.5 2.5 0
2.5 2.5 0
2.5 2.5 10
-2.5 2.5 10
- You will use fog (in the computer graphics sense) to make objects further away appear darker using glFogi(GL_FOG_MODE, GL_LINEAR);
glFogi(GL_FOG_START, <where it starts close to you>);
glFogi(GL_FOG_END, <where things are completely obscured in the distance>);
glEnable(GL_FOG);
- You will map a modulated texture onto the floor and a different texture onto the walls to make the scene look more realistic. With a modulated texture the lighting will affect the texture makign the scene look more realistic than with a decal texture where the light would be ignored. Here you will get to use glTexImage2D to define the texture, glTexParameterf to define how to map the wrap the texture, glTexEnvf to set the texture to be modulated, glEnable to turn on texture mapping and glTexCoord2f to map specific texture coordinates onto specific vertices. You can generate some simple texture maps yourself through code, or you can convert existing textures into images that your program can read in. You can find some textures here: http://www.pixelfoundry.com/bgs.html. I'm not saying these are the most appropriate but its a starting point. To use existing textures such as these you will probably want to convert the texture into raw RGB bytes rather than in some image format such as PNG, TIFF , JPG etc - it makes reading the texture in easier. Photoshop, XV, etc can do this quite easily. You may also want to start with a blank and white bitmapped texture, or greyscale texture before moving onto a dull colour image.
- The older style 'T' teleporters in the maze will be upgraded to directional teleporters. The data file can now have 1, 2, 3, 4, 5, 6, 7, 8, 9, 0 in it. Stepping on the '1' will teleport you to the '2'. Stepping on the '3' teleports you to the '4', etc. Stepping on an odd number moves you to the appropriate even number. Stepping on the even number does nothing since its a receiver. This means that the maze can now be made up of several distinct blocks of rooms that are only linked by teleporters.
- You will use the SGI-specific sound routines to make your program a little more interesting by have some sounds triggered on various events. The simplest way to do this is to have the system execute 'sfplay <soundfile> &' when an appropriate event occurs. You can find some sample sound files at http://www.partnersinrhyme.com/contents/contentssfx.html. Again I'm not endorsing the sounds, just giving a pointer. Soundeditor on the O2s can be used to convert file formats. Hint - you should probably have all of your sounds at the same sampling rate.
- You will give the user the ability to switch from their first-person view to a map view (top down view) of the maze centered on their current position. The map should contain some representation of the user at that spot. The user can still navigate (using the i, j, l keys) and the map should update appropriately. That is, the user can still play the game in map mode.
- You will also add in another three characters. Since this is a modified Berzerk, we will call each of them Otto. An Otto is a sphere that flies through the maze 4 feet above the ground and bounces off the walls of the maze similar to the wall bouncing you have implemented before. Each Otto should move at about the same speed as the user. If an Otto flies over an active teleporter (one of the odd numbers) then he will teleport. Unlike Berzerk, you will be chasing them. If you catch one of them, it will reappear somewhere else and continue to bounce around the maze. In Map mode the Ottos should appear on the map and the user should be able to watch them moving around.
- A textual display will show how many times you have caught Otto, and how many orbs the user has picked up.
- You should design an intersting maze for the user to wander around in.
- Some of the features added here require hardware acceleration to work at interactive speeds. For modern graphics cards (within the last year or two) they should not be a problem. For older machines (like the O2s) it might be. You should allow the user to interactively turn on/off the textures and the fog. The game should still look good and be playable without the textures and fog.
Note that this writeup is intentionally not giving you all the details on how to go about doing all of these various things. There are several nice links on the web pages to the man pages for the various OpenGL functions, and the Red Book online, and sample code so you should do some research before you start coding on how to apply the various routines. The basic goal here is to come up with a game that is visually interesting while still being quite playable on the O2s.
Also note that while creativity is encouraged on this assignment that does not mean that you should grab textures or sounds from published sources without their permission. Creating your own sounds or textures is highly encouraged, and using public domain images and sounds are fine as long as you cite the source.
As usual, your program should be well commented and be a good example of literate programming.
As with the previous assignments your program will be submitted electronically and you need to be sure that it compiles and runs on the SGI O2s in the EECS lab.
Most of the enhancements in this assignment are independant of each other, but you should switch over to the filled polygonal maze first and then upgrade the graphics.
Again, I would also highly recommend that you understand the code you are writing - you never know when you might need to reproduce it (hint hint).
|
|
 |
[jump to top of page]
The above document was last modified on 2004-04-18 21:22:07;
page last updated on 2010-07-02 at 21:22:34.
Document size: 10696 bytes, plus related data up to 96.0 kbytes.
|
Contents:
|